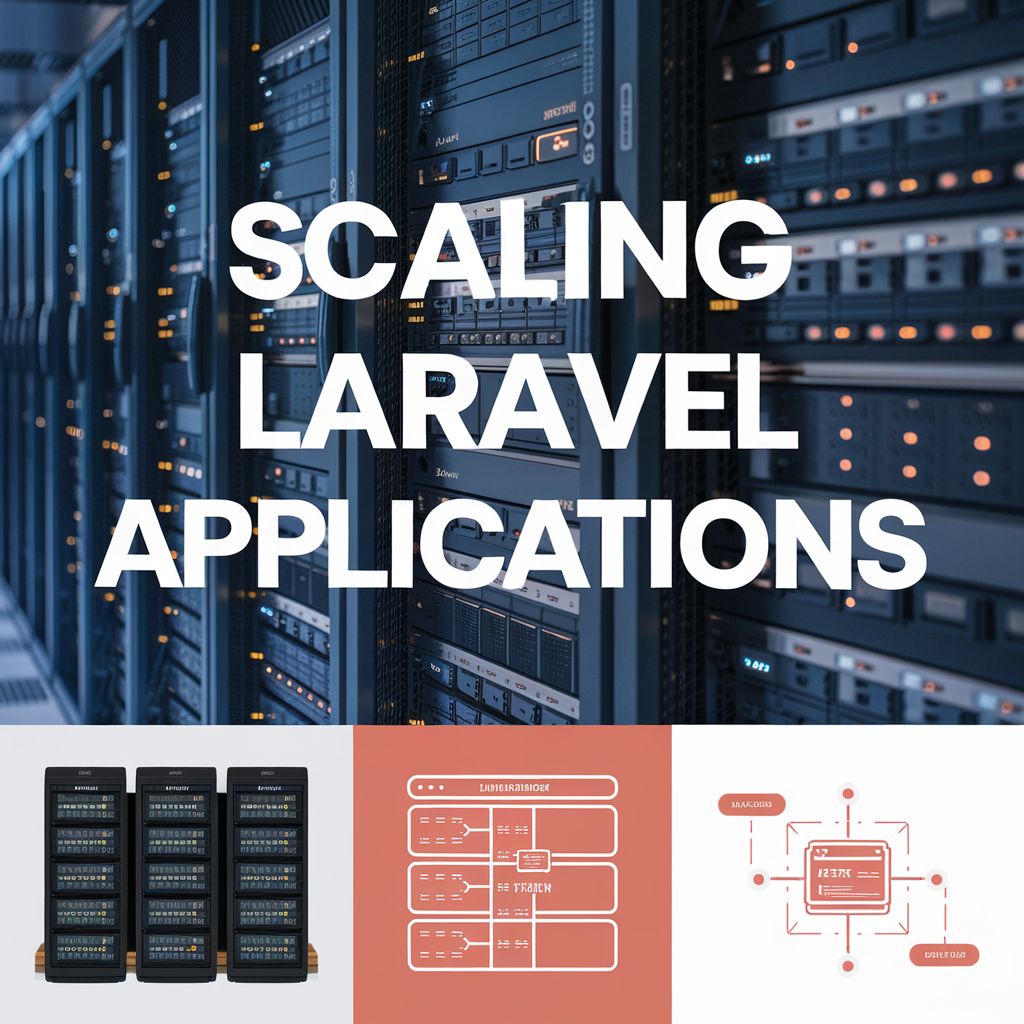
Laravel is a popular PHP tool for building websites. Developers like it because it’s easy to use and has helpful features. Scaling Laravel Applications But as your app gets more users, it needs to handle the extra traffic or it might become slow or crash.
In this guide, we’ll look at different ways to make your Laravel app handle more traffic and perform better. We’ll talk about things like the database, servers, caching, and other ways to improve performance.
What Are Some Effective Strategies For Scaling Laravel Applications To Handle Increased User Traffic?
1. Optimize Your Code
Write clean and efficient code to reduce unnecessary database queries. Use techniques like eager loading to fetch data more efficiently. Make sure your app runs smoothly by profiling and optimizing slow parts of the code.
2. Database Scaling
Distribute the database load across multiple servers using horizontal scaling. Use read replicas to offload read queries and improve performance. Consider using NoSQL databases like MongoDB for handling large, flexible data more efficiently.
3. Use Caching
Cache frequently accessed data to reduce the need for repeated database queries. Tools like Redis or Memcached allow fast data retrieval, speeding up response times. Store data in the cache instead of querying the database for the same information multiple times.
4. Asynchronous Processing
Move heavy tasks like report generation to background queues using Laravel’s job system. This keeps the app responsive and ensures users don’t experience long wait times. Laravel Horizon helps you manage and monitor queued jobs efficiently.
5. Server Scaling
Scale your application by adding more servers behind a load balancer to handle higher traffic. This spreads the user load across multiple servers and avoids bottlenecks. It also ensures that your app remains available even during traffic spikes.
6. Content Delivery Network (CDN)
Use a CDN to cache and serve static files like images, CSS, and JavaScript. This reduces the load on your server and speeds up content delivery by serving files from servers closer to users. CDNs also improve site performance by distributing content worldwide.
Use Database Scaling Techniques:
1. Database Horizontal Scaling
Horizontal scaling spreads the database load across multiple servers. This allows you to add more servers as your user base grows. Laravel simplifies this process with database replication and load balancing to manage traffic.
2. Use NoSQL Databases
NoSQL databases like MongoDB or Cassandra are built to handle large volumes of data and scale easily. They are ideal for flexible, high-volume applications. Laravel allows easy integration with these databases, making the switch smooth.
3. Add Read Replicas
Read replicas help share the load of database read requests, improving performance. By offloading read operations, the main database can focus on writing data. Laravel supports read replicas, reducing the strain on the primary database server.
4. Use Caching
Caching stores frequently accessed data in fast memory, reducing the need to query the database repeatedly. This boosts performance by speeding up data retrieval. Laravel integrates well with Redis, making caching simple and efficient.
Optimize Your Laravel Code:
To optimize your Laravel code, start by following best coding practices to ensure your code is clean, efficient, and easy to maintain. Enable OPcache for faster PHP execution by caching compiled code, which reduces the need for recompiling with every request.
Use profiling tools to detect and fix performance issues in your application, focusing on the slowest parts. To avoid N+1 query problems, utilize eager loading rather than lazy loading to prevent unnecessary database queries. For large result sets, paginate your queries instead of loading all records at once, which reduces memory usage and improves response time.
Optimize your database queries by using proper indexing, selecting only the necessary fields, and avoiding redundant joins. For time-consuming tasks like data processing or generating reports, move them to background jobs using Laravel’s queue system to keep your application responsive. Lastly, cache frequently accessed data with tools like Redis to avoid repetitive database queries, speeding up data retrieval.
What Does It Mean To Scale Your Laravel Servers?
1. Increase Server Capacity:
Scaling your Laravel servers means adding more resources (such as memory, storage, or processing power) to handle more traffic and users.
2. Distribute Traffic:
It involves using techniques like load balancing to distribute incoming user traffic across multiple servers, preventing any single server from becoming overloaded.
3. Improve Performance and Reliability:
Scaling ensures that your application performs well even under high traffic and remains available, minimizing downtime and lag for users.
4. Handle Growth:
As your app’s user base grows, server scaling helps ensure the infrastructure can support the increased demand without affecting the user experience.
5. Adaptable Infrastructure:
Server scaling allows your infrastructure to adapt to changing demands, whether through vertical scaling (upgrading existing servers) or horizontal scaling (adding more servers).
Implement Application Caching:
To implement application caching in Laravel, start by caching frequently accessed data to reduce database load. Use caching systems like Redis or Memcached for faster data retrieval. You can cache full pages, especially for content that doesn’t change frequently, to speed up response times.
Cache API responses to avoid repetitive database queries and improve performance. For complex and resource-intensive processes, such as sorting or generating recommendations, store the results in the cache. Laravel makes caching simple with built-in support for various caching drivers. Additionally, using a CDN to cache static assets like images and scripts can greatly improve load times for users.
FAQs:
Why is auto scaling important?
Auto scaling automatically adjusts server capacity based on real-time demand. It ensures that your application has enough resources during traffic surges and reduces costs during low-traffic periods by scaling down.
How to scale PHP applications?
To scale PHP applications, use horizontal scaling by adding more servers behind a load balancer to distribute traffic. Optimize performance with caching, database replication, and asynchronous processing to handle increased user demand efficiently.
How many requests per second can Laravel handle?
The number of requests Laravel can handle depends on server resources and optimization techniques like caching and load balancing. With proper scaling and optimization, it can manage hundreds to thousands of requests per second.
What are the key strategies for scaling Laravel applications?
Key strategies include optimizing code, using database scaling techniques like read replicas and NoSQL databases, implementing caching with Redis, and scaling servers horizontally using load balancers.
How do I know when my Laravel application needs scaling?
You’ll need to scale when you notice slow response times, increased server load, or when your app starts experiencing downtime due to traffic spikes or growing user demand.
Conclusion:
Scaling a Laravel app involves improving code, optimizing the database, using caching, scaling servers, and setting up auto-scaling. Using the right tools and strategies, your app can easily handle millions of users without performance issues.